![[Swift] continue, fallthrough-switch, where-for, enumerated, zip, indices](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FPPf8K%2FbtrI2YyYMX2%2FZUMz2db1mkcLz7FJIIBxq1%2Fimg.png)
[Swift] continue, fallthrough-switch, where-for, enumerated, zip, indicesLanguage/Swift2022. 8. 7. 23:11
Table of Contents
Continue
: 반복문 내에서 그 지점을 건너뛰고 계속 진행한다는 의미입니다.
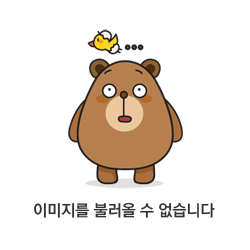
outerloop: for i in 1...3 {
innerloop: for j in 1...3 {
if j == 3 {
continue outerloop
}
print("i = \(i), j = \(j)")
}
}
레이블이 있는 반복문으로 innerloop이 아닌 outerloop으로 보냅니다.
fallthrough가 있는 switch 문
: case 값이 switch 식과 일치하지 않아도 제어는 다음 case로 진행합니다.
let dayOfWeek = 4
switch dayOfWeek {
case 1:
print("Sunday")
case 2:
print("Monday")
case 3:
print("Tuesday")
case 4:
print("Wednesday")
fallthrough
case 5:
print("Thursday")
case 6:
print("Friday")
case 7:
print("Saturday")
default:
print("Invalid day")
}
case 5까지 진행되어 "Thursday"도 출력하게 됩니다.
where가 있는 for-in문
: for-in루프에서 필터를 구현하는 데 사용됩니다. 즉, where절의 조건이 반환 true되면 루프가 실행됩니다.
// remove Java from an array
let languages = ["Swift", "Java", "Go", "JavaScript"]
for language in languages where language != "Java"{
print(language)
}
필터 같이 "Java"를 제외하고 language 배열의 요소들이 출력됩니다.
역순으로 for-in문
: stride 사용, reverse 사용
for i in stride(from: 5, to: 0, by: -1) {
print(i)
}
// 5 4 3 2 1 출력
for i in stride(from: 5, through: 0, by: -1) {
print(i)
}
// 5 4 3 2 1 0 출력
for i in (0...5).reversed() {
print(i)
}
// 5 4 3 2 1 0 출력
enumerated (참고)
: Returns a sequence of pairs (n, x), where n represents a consecutive integer starting at zero and x represents an element of the sequence. (0부터 연속된 숫자 n, 요소 x를 반환합니다)
let names: Set = ["Sofia", "Camilla", "Martina", "Mateo", "Nicolás"]
var shorterIndices: [Set<String>.Index] = []
for (i, name) in zip(names.indices, names) {
if name.count <= 5 {
shorterIndices.append(i)
}
}
for i in shorterIndices {
print(names[i])
}
zip
: 두 개의 기본 시퀀스로 구성된 쌍의 시퀀스를 만듭니다.
let words = ["one", "two", "three", "four"]
let numbers = 1...4
for (word, number) in zip(words, numbers) {
print("\(word): \(number)")
}
전달된 두 시퀀스의 길이가 다른 경우 결과 시퀀스는 더 짧은 시퀀스와 길이가 같습니다.
Array.indices
: 0에서 배열의 크기까지 계산
for i in movies.indices.reversed() {
...
}
for i in (0 ..< movies.count).reversed() {
...
}
두 개의 코드는 동일하게 작동합니다
'Language > Swift' 카테고리의 다른 글
swift computed property 연산 프로퍼티 (0) | 2022.08.23 |
---|---|
swift nested function (0) | 2022.08.22 |
swift defer 블록 (0) | 2022.08.22 |
[Swift] guard 문 (0) | 2022.08.07 |
[Swift] C에 대해서 알면 좀 쉬울지도? swift 문법 (0) | 2022.08.02 |
@jaewpark :: 코스모스, 봄보다는 늦을지언정 가을에 피어나다
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!