![[Swift] [leetcode] Palindrome Number ... etc](https://img1.daumcdn.net/thumb/R750x0/?scode=mtistory2&fname=https%3A%2F%2Fblog.kakaocdn.net%2Fdn%2FxShhu%2FbtrI8RyacIa%2FIT6RN5UUvae6m1e3hSKMi0%2Fimg.png)
문제 Palindrome Number
Palindrome Number - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
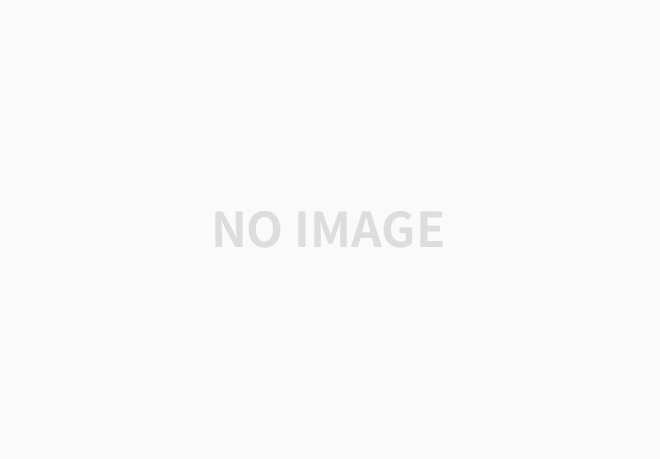
Constraints(제약 조건)의 범위의 숫자가 들어오는데, 완벽한 대칭이 되는 숫자의 경우에만 true 값을 반환하면 됩니다.
1. 숫자를 문자열로 변환
2. 문자열의 index값을 이용하여, 앞과 뒤를 비교
3. 2번을 문자열의 사이즈 1/2 까지만 반복문 실행
swift 문자열 index 접근은 c와 같이 접근을 하지 못하고 c++의 반복자와 같이 사용을 해야합니다.
(참고 :2022.07.27 - [42 Seoul] - [42Seoul] CPP08 STL)
Manipulating Indices (참고)
startIndex : The position of the first character in a nonempty string
endIndex : A string’s “past the end” position—that is, the position one greater than the last valid subscript argument.
index(before: String.Index) : Returns the position immediately before the given index.
index(after: String.Index) : Returns the position immediately after the given index.
index(String.Index, offsetBy: String.IndexDistance) : Returns an index that is the specified distance from the given index.
위에서 받은 index를 이용해서 값에 접근할 수 있습니다
var start = string.startIndex
string[start] // 문자열 첫번째 값
Swift index를 이용해서 푼 코드는
class Solution {
func isPalindrome(_ x: Int) -> Bool {
let stringInt:String = String(x)
var start = stringInt.startIndex
var end = stringInt.index(before: stringInt.endIndex)
while start < end {
if stringInt[start] != stringInt[end] {
return false
}
start = stringInt.index(after: start)
end = stringInt.index(before: end)
}
return true
}
}
while 조건문에서 start 와 end가 같다면
- x는 1의 자리수
- x의 길이가 홀수
위의 경우에는 true 값이기 때문에 바로 return을 하면 됩니다.
문제 Longest Substring Without Repeating Characters
Longest Substring Without Repeating Characters - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
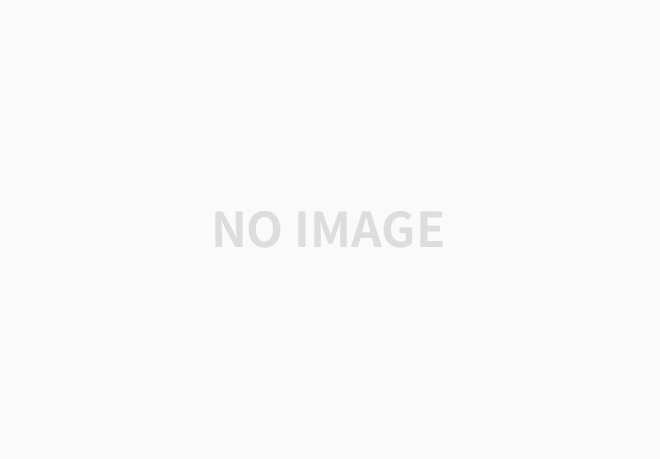
주어진 문자열에서 중복되지 않는 가장 긴 문자열을 구하는 문제입니다.
class Solution {
func lengthOfLongestSubstring(_ s: String) -> Int {
var longest = 0, startIndex = 0
var charMap: [Character: Int] = [:]
for (index, char) in s.enumerated() {
if let foundIndex = charMap[char] {
startIndex = max(foundIndex + 1, startIndex)
}
longest = max(longest, index - startIndex + 1)
charMap[char] = index
}
return longest
}
}
class Solution {
func lengthOfLongestSubstring(_ s: String) -> Int {
let str = Array(s.unicodeScalars)
var maxCount = 1
var startIndex = 0
guard str.count > 0 else {
return 0
}
guard str.count > 1 else {
return 1
}
for index in 1..<str.count {
if let repIndex = str[startIndex..<index].firstIndex(where: { $0 == str[index] }) {
maxCount = max(maxCount, index - startIndex)
startIndex = repIndex + 1
}
}
maxCount = max(maxCount, str.count - startIndex)
return maxCount
}
}
위 코드는 Map을 이용해서 글자를 통해 index를 계속 갱신을 하면서, 시작 포인트를 변경해줍니다.
그리고 제일 긴 길이를 변수로 가지고 있으면서 시작포인트에서 지금 까지의 길이를 확인하여 값을 갱신합니다.
두 번째의 코드는 Array로 firstIndex를 이용해서 Starting 포인트를 갱신하여 길이를 확인합니다.
문제 Zigzag Conversion
Zigzag Conversion - LeetCode
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
leetcode.com
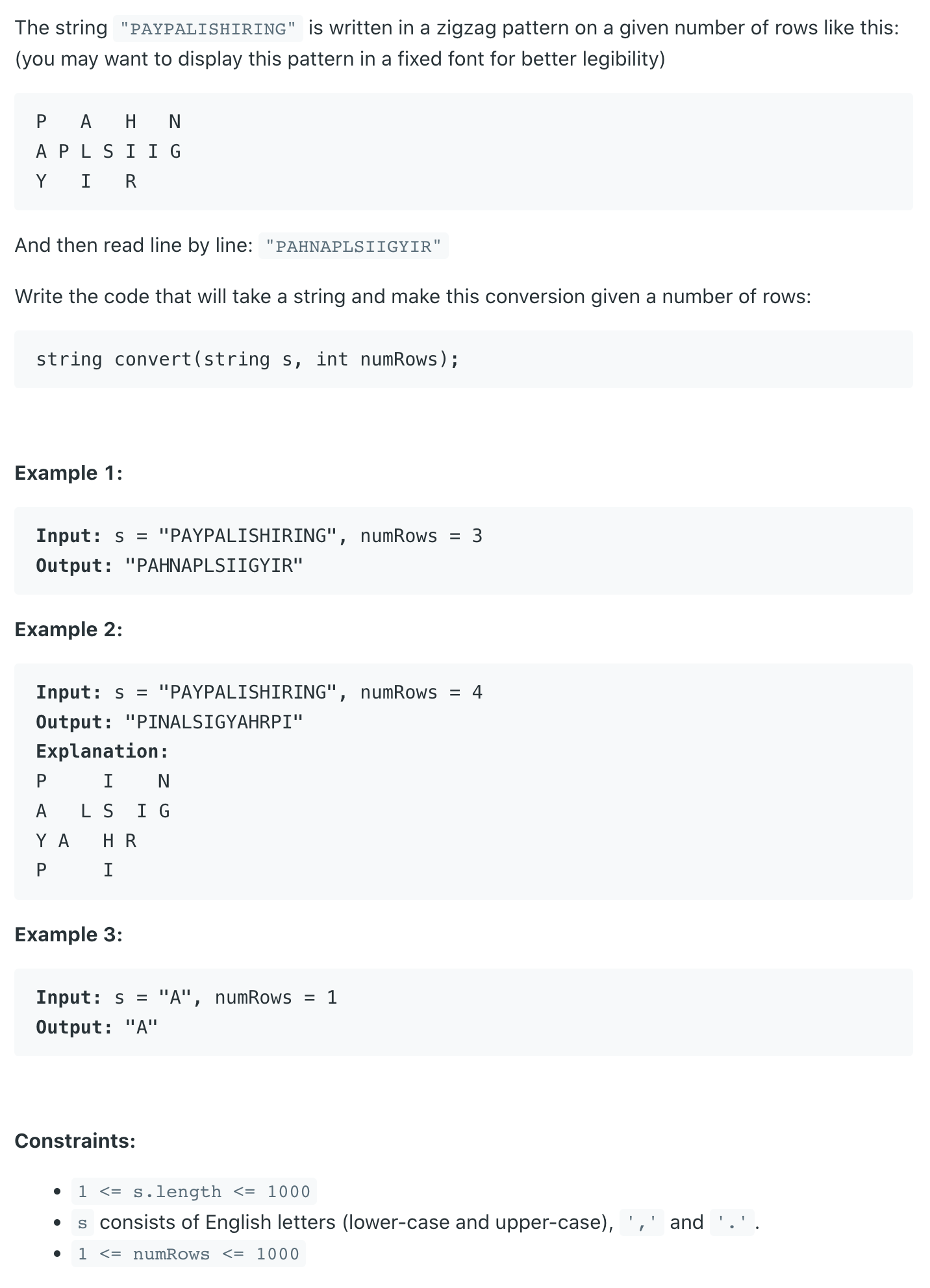
지그재그로 표현을 했을 때, 위와 같은 그림대로 그려지고 첫 줄부터 읽게 되었을 때 문자열들을 합쳐서 반환해야 합니다.
numRows에 따라서 규칙이 발생되는 데 3일 때에는 [0, 1, 2, 1], 4일 때에는 [0, 1, 2, 3, 2, 1] 의 규칙이 반복되어 글자가 저장되는 걸 발견할 수 있습니다. 그럼 numRows에 맞춰 2차원 배열을 생성하고 글자를 넣어주면 됩니다.
class Solution {
func convert(_ s: String, _ numRows: Int) -> String {
guard numRows > 1 else { return s }
var rows: [[Character]] = []
var res = ""
for _ in 0..<numRows { rows.append([]) }
for (i, c) in s.enumerated() {
let index = i % (2 * numRows - 2)
if index < numRows {
rows[index].append(c)
} else {
rows[2 * numRows - index - 2].append(c)
}
}
for row in rows {
for c in row { res += String(c) }
}
return res
}
}
index구하는 식에서 (2 * numRows - 2) 는
numRows이 3일 때 4개, 4일 때 6, 5는 8 이렇게 되는 규칙을 찾아 공식으로 만들어 줍니다.
위에서 언급했던 3 = [0, 1, 2, 1], 4 = [0, 1, 2, 3, 2, 1] ... 진행이 될 때
numRows보다 작은 index는 그대로 넣어주면 되고, index가 numRows보다 큰 경우에는 [2 * numRows - index - 2]를 하게되면 공식 값에 해당하는 배열에 c 값을 넣을 수 있습니다.
사용된 guard문과 enumerated는 위에서 사용된 문법을 정리하여 따로 글로 쓸 예정입니다
'자료구조와 알고리즘 > 알고리즘' 카테고리의 다른 글
[Swift] 백준 16173번: 점프왕 쩰리 - BFS (0) | 2024.03.08 |
---|---|
[Swift] [leetcode] Implement strStr ... etc (0) | 2022.08.09 |
[Swift] [프로그래머스 1단계] 키패드 누르기 (0) | 2022.08.06 |
[Swift] [프로그래머스 1단계] 체육복 (0) | 2022.08.04 |
9461 백준 문제풀이 (C언어) (0) | 2021.04.29 |
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!